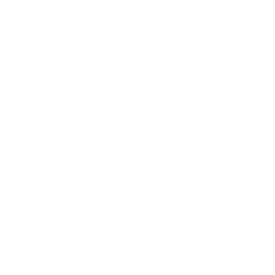
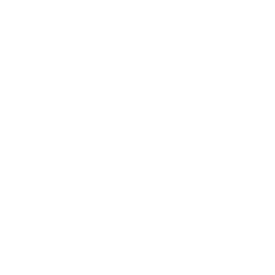
The Roman empire had one of the greates armies in history. It was big, and large, and vast. In fact, it was so big that only one person in the whole Empire could count that high. The problem was that he learned counting in the Sofia Math Highshcool, where only Arabic numbers were taught. So every time the Emperor asked “How big is our army?” the reply was meaningless to him.
Help him by writing a method number_to_roman
that converts from an Arabic number to
a roman literal. Also, keep it as short as possible as the Emperor is quite slow with the
keyboard and the Empire might fall before he’s finished typing it in.
Examples:
number_to_roman(1) => 'I' number_to_roman(13) => 'XIII' number_to_roman(2016) => 'MMXVI'
The lower the score - the better. It is the shortest solution that wins.
Nickname: Rosa Score: 120
def number_to_roman(n) %w(M CM D CD C XC L XL X IX V IV I).zip(%w(1000 900 500 400 100 90 50 40 10 9 5 4 1)).map{ |l,v| a, n=n.divmod v.to_i l*a }*'' end
Nickname: janosch-jo Score: 125
def number_to_roman n 'M1000CM900D500CD400C100XC90L50XL40X10IX9V5IV4I1' .scan(/(\D+)(\d+)/) .map { |k, v| c, n = n.divmod v.to_i k*c } * '' end
Nickname: babi Score: 136
def number_to_roman(n) {M:1000,CM:900,D:500,CD:400,C:100,XC:90,L:50,XL:40,X:10,IX:9,V:5,IV:4,I:1}.map{|l,v|a,n=n.divmod v;l.to_s*a}.join end
Nickname: timrogers Score: 138
R = { M: 1e3, CM: 900, D: 500, CD: 400, C: 100, XC: 90, L: 50, XL: 40, X: 10, IX: 9, V: 5, IV: 4, I: 1 } def number_to_roman i R.map{|(l,n)|q,r = i.divmod n i = r "#{l}"*q }*"" end
Nickname: Joskov Score: 145
def f n o = [1, 4, 5, 9, 10, 40, 50, 90, 100, 400, 500, 900, 1000] i = (o.find_index { |x| x > n } || 0) - 1 n > 0 ? %w(I IV V IX X XL L XC C CD D CM M)[i] + f(n - o[i]) : '' end alias number_to_roman f
Nickname: ben_ Score: 151
def number_to_roman(number) {M:1000,CM:900,D:500,CD:400,C:100,XC:90,L:50,XL:40,X:10,IX:9,V:5,IV:4,I:1}.map{|l,v|a,number=number.divmod v;l.to_s*a}.join end
Nickname: J-_-L Score: 157
def number_to_roman n s="" %w[M C X I].zip([n/1000,n/100,n/10,n]){|l,m|s+=l.*m%10} [%w[I V X],%w[X L C],%w[C D M]].map{|a,b,c|s.gsub!a*9,a+c s.gsub!a*5,b s.gsub!a*4,a+b} s end
Nickname: fp Score: 160
def number_to_roman(n) r = %w[1000 900 500 400 100 90 50 40 10 9 5 4 1].map(&:to_i).zip(%w[M CM D CD C XC L XL X IX V IV I]) z = "" while n > 0 && r.any? k, v = r.first n - k >= 0 ? (z << v; n -= k) : r.shift end z end
Nickname: Luca Score: 162
def number_to_roman n,a="" n==0 ? a : ( k,v=([1000]+[100,10,1].flat_map{|x| [9,5,4,1].map{|y| x*y} }).zip(%w[M CM D CD C XC L XL X IX V IV I]).find {|k| k[0]<=n} number_to_roman n-k, a+v) end
Nickname: Luke Score: 165
def number_to_roman(n,a="") n==0 ? a : ( k,v=([1000]+[100,10,1].flat_map{|x| [9,5,4,1].map{|y| x*y} }).zip(%w[M CM D CD C XC L XL X IX V IV I]).find {|k, v| k<=n} number_to_roman(n-k, a+v)) end
Nickname: Lucaong Score: 169
def number_to_roman(n,a="") return a if n==0 k,v=([1000]+[100,10,1].flat_map{|x| [9,5,4,1].map{|y| x*y} }).zip(%w[M CM D CD C XC L XL X IX V IV I]).find {|k,v| k<=n} number_to_roman(n-k, a+v) end
Nickname: Joan Score: 174
def number_to_roman(x) [3,2,1,0].map{|i|n=x.to_s.rjust(4,'0')[3-i].to_i;g=%w(I V X L C D M _ _).slice(i*2,i*2+3);n>=0&&n<4?g[0]*n: n==4?g[0]+g[1]: n==9?g[0]+g[2]:g[1]+g[0]*(n-5)}.join end
Nickname: tpavel Score: 200
C = [ ['M', 1000], ['CM', 900], ['D', 500], ['CD', 400], ['C', 100], ['XC', 90], ['L', 50], ['XL', 40], ['X', 10], ['IX', 9], ['V', 5], ['IV', 4], ['I', 1] ] def number_to_roman(n) r = '' C.each do |s| while n >= s[1] r += s[0] n -= s[1] end end r end
Nickname: Ben Score: 201
def number_to_roman(number) { "M" => 1000, "CM" => 900, "D" => 500, "CD" => 400, "C" => 100, "XC" => 90, "L" => 50, "XL" => 40, "X" => 10, "IX" => 9, "V" => 5, "IV" => 4, "I" => 1 }.map do |ltr, val| amt, number = number.divmod(val) ltr * amt end.join end
Nickname: M. Valenzuela Score: 218
def number_to_roman(number) ''.tap do |r| m={1000=>'M',900=>'CM',500=>'D',400=>'CD',100=>'C',90=>'XC',50=>'L',40=>'XL',10=>'X',9=>'IX',5=>'V',4=>'IV',1=>'I'} while(number!=0) do k,v=m.find{|k,_|k<=number} number-=k r<<v end end end
Nickname: xijo Score: 237
def number_to_roman(n) h = { M:1000, D:500, C:100, L:50, X:10, V:5, I:1 } s = h.map{ |k, v| c, n = n.divmod(v) k.to_s*c } * '' h.keys.each_with_index { |k, i| before = h.keys[i-1] s.sub!(/#{before}#{k}{4}/, "#{k}#{h.keys[i-2]}") s.sub!(/#{k}{4}/, "#{k}#{h.keys[i-1]}") } s end